jQuery セレクタ フォームのセレクタ
jQueryでは、フォーム要素に対しても、様々なセレクタが用意されています。:inputは、フォーム内のすべてのコントロールにマッチします。:inputは、フォーム内のすべてのコントロールにマッチします。「input type=”text”」や「input type=”checkbox”」といった、タグに「input」を含む要素だけでなく、select、textareaにもマッチします。
:text、:checkbox、radio、:password、:file、:image、:button、;submit、:resetは、それぞれ対応するフォームコントロールすべてにマッチします。:checkedはチェックボックスとラジオボタンに有効なセレクタで、チェックされている要素にマッチします。:selectedはオプションリストに有効なセレクタで、選択されたoption要素にマッチします。multiple=”multiple”で複数選択可能なオプションリストでは、選択されていれば複数のoption要素にマッチします。
:disabledはdisabled=”disabled”で無効状態になっている要素にマッチします。disabled属性がフォームコントロールだけを対象にしているわけではありません。$(‘:disabled’)だけのセレクタは、<p disabled=”disabled”>などの要素にもマッチします。フォーム要素を対象にしたい場合は、$(‘input:disabled’)とします。
書式
$(':checked')
$(':selected')
$(':disabled')
$(':enabled')
$(':focus')
$(':button')
$(':checkbox')
$(':file')
$(':image')
$(':input')
$(':password')
$(':radio')
$(':reset')
$(':submit')
$(':text')
$('input:checked')
$('select option:selected')
$('input:disabled')
$('input:enabled')
$(':focus')
$('input:button')
$('input:checkbox')
$('input:file')
$('input:image')
$(':input')
$('input:password')
$('input:radio')
$('input:reset')
$('input:submit')
$('input:text')
サンプル
1、チェックが入ったものを取得。サンプルでは「click」ボタンをクリックするとチェックボックス、ラジオボタンでチェックが入ったものを取得して赤枠で囲むようにしています。「:checked」はチェックされた要素を取得するものであり、チェックボックスだけでなくラジオボタンのチェックも選択されます。
<body>
チェックボックス:
<label><input name="checkBox" type="checkbox">A</label>
<label><input name="checkBox" type="checkbox">B</label>
<label><input name="checkBox" type="checkbox" checked="checked">C</label>
<br>
<br>
<label><input name="radioButton" type="radio" checked="checked">A</label>
<label><input name="radioButton" type="radio">B</label> <label><input name="radioButton" type="radio">C</label>
<br>
<br>
<button>click</button>
</body>
<script>
$("button").on('click', function() {
$(":checked").parent().css("border", "1px solid red");
});
</script>
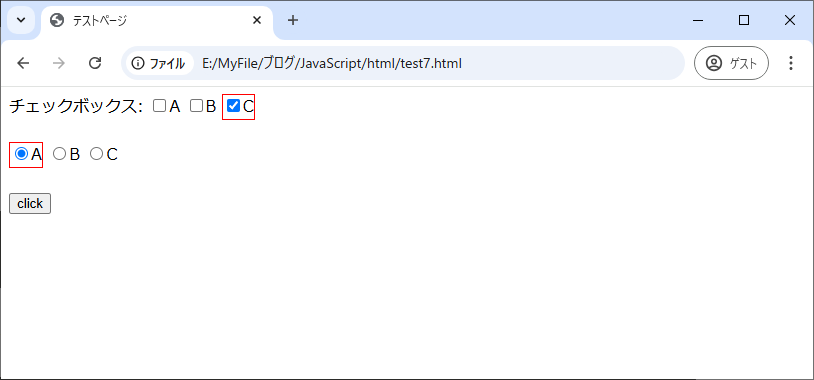
2、選択されている要素を取得。サンプルでは「click」ボタンをクリックすると選択されている要素をテキストとして表示します。「:selected」はselect要素で選択されている要素を種痘します。対応している要素はoption要素だけのため、チェックボックスやラジオボタンで選択されている要素を取得したい場合は「:checked」を使用しましょう。
<head>
<meta charset="UTF-8">
<title>テストページ</title>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<style>
div { color: red; }
select { font-size: 13px; }
</style>
</head>
<body>
<select name="garden" multiple="multiple">
<option>東京</option>
<option>埼玉</option>
<option>群馬</option>
<option>茨木</option>
<option>栃木</option>
<option>千葉</option>
<option>神奈川</option>
</select>
<button>click</button>
<div></div>
</body>
<script>
$("button").on('click', function() {
var str = $(":selected").text();
$("div").text(str + 'が選択されています');
});
</script>
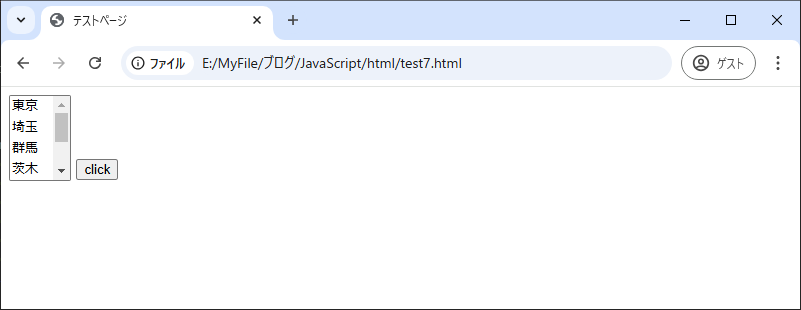
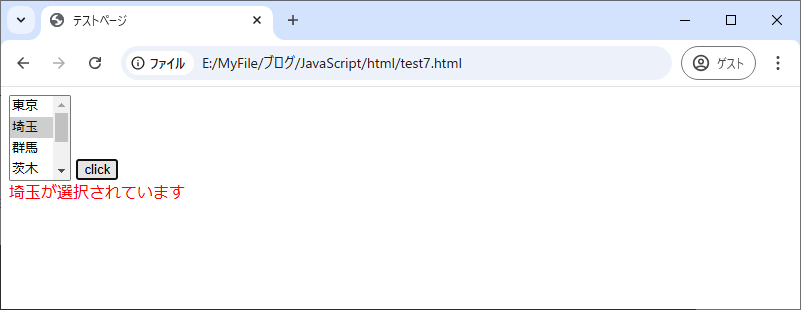
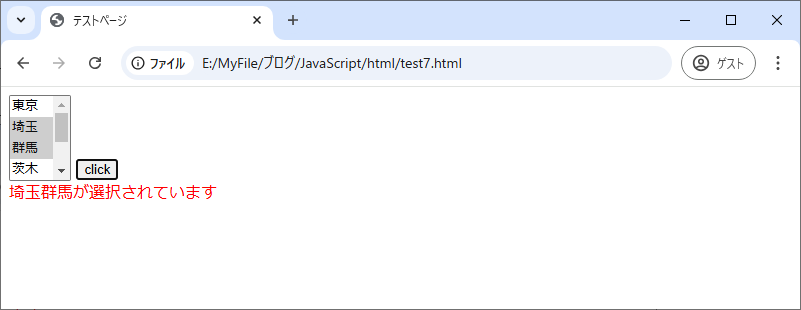
3、無効状態の要素を取得。「:disabled」は無効状態のフォームの要素を選択します。サンプルでは「disabled」が設定され、無効になっている要素に赤枠を付けています。また、「:enabled」はこれとは反対に有効になっている要素を取得します。
<body>
<input name="mail" disabled="disabled">
<input name="id">
<br>
<textare name="text1" rows="4" cols="40" disabled="disabled">テキストエリア</textare>
<textare name="text2" rows="4" cols="40" >テキストエリア</textare>
</body>
<script>
$(":disabled").css("border","1px solid red");
</script>
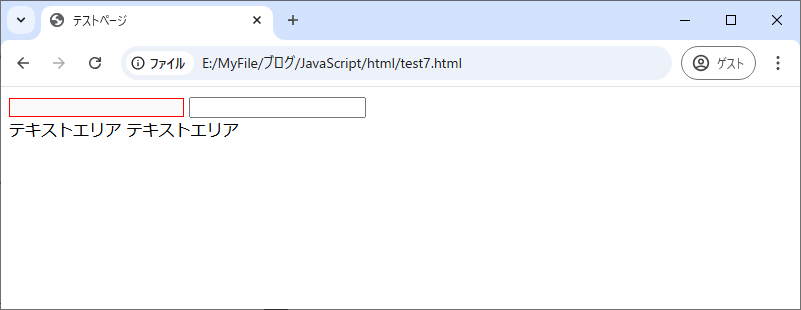