jQuery 「イベント」マウスカーソルの要素への出入り
mouseover()とmouseenter()イベントは、要素にマウスカーソルが入った時に発生します。同様に、マウスカーソルが要素から出たとき、mouseout()、mouseleave()イベントが発生します。mouseout()とmouseleave()、mouseout()とmouseleave()の違いは、ともに要素の関係とイベントバブリングに関するものです。それぞれの前者は要素同士の親子関係を考慮しませんが、後者は親子関係に基づいてイベントの発生を制御します。例えば要素Aと要素Bが親子関係にあった場合、AからB、BからAへのカーソルの移動時に常にmouseover()、mouseenter()イベントハンドラがコールされます。一方、子である要素Bから要素Aへのカーソルの移動時、要素Aのmouseenter()イベントは発生しません。親である要素Aから子の要素Bへの移動時も、要素Aのmouseleave()イベントは発生しません。
対象となるHTML要素には制限はなく、どの要素にでも、これらのイベントハンドラを結び付けられます。引数無しのメソッドは、それぞれ「trigger(‘mouseover’)」「trigger(‘mouseout’)」「trigger(‘mouseenter’)」「trigger(‘mouseleave’)」の短縮形です。
書式
$(対象要素).on('mouseover', function(e){処理…})
$(対象要素).on('mouseout', function(e){処理…})
$(対象要素).on('mouseenter', function(e){処理…})
$(対象要素).on('mouseleave', function(e){処理…})
$(対象要素).trigger('mouseover')
$(対象要素).trigger('mouseout')
$(対象要素).trigger('mouseenter')
$(対象要素).trigger('mouseleave')
$(対象要素).mouseover()
$(対象要素).mouseout()
$(対象要素).mouseenter()
$(対象要素).mouseleave()
$('p').mouseover(function(e){
alert('mouse over');
})
$('p').mouseout(function(e){
alert('mouse out');
})
$('p').mouseenter(function(e){
alert('mouse enter');
})
$('p').mouseleave(function(e){
alert('mouse leave');
})
$('div').trigger('mouseover');
$('div').trigger('mouseout');
$('div').trigger('mouseenter');
$('div').trigger('mouseleave');
$('div').mouseover();
$('div').mouseout();
$('div').mouseenter();
$('div').mouseleave();
サンプル
1、マウスの出入りを検知する(enter/leave)
<html>
<head>
<meta charset="UTF-8">
<title>テストページ</title>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<style>
.mouseground{
width: 400px;
height: 400px;
background-color: rgb(96, 100, 99);
}
.mousecage{
width: 200px;
height: 200px;
background-color: rgb(69, 143, 124);
}
</style>
</head>
<body>
<div class="main">
<div class="header">
<h1> サンプル </h1>
</div>
<div class="content">
<h2>マウスの移動範囲</h2>
<div class="mouseground">
<div class="mousecage">
</div>
</div>
<div class="desc"></div>
</div>
<div class="footer">
<hr>
<p class="copyright">2019 xxxx all rights reserved.</p>
</div>
</div>
<script>
$(document).ready(function(){
$('.mouseground').on('mouseenter', function(){
$('.desc').append('入りました(グレー)<br>');
}).on('mouseleave', function(){
$('.desc').append('外れました(グレー)<br>');
});
$('.mousecage').on('mouseenter', function(e){
$('.desc').append('入りました(緑)<br>');
}).on('mouseleave', function(e){
$('.desc').append('外れました(緑)<br>');
});
});
</script>
</body>
</html>
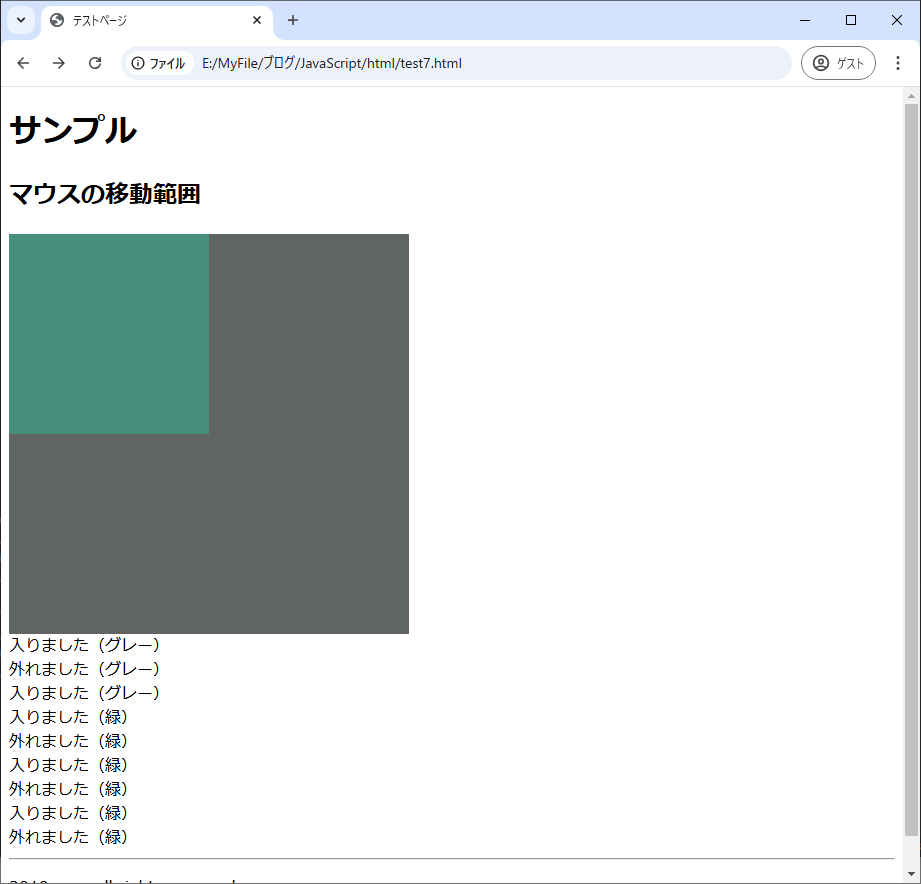
2、マウスの出入りを検知する(over/out)
<html>
<head>
<meta charset="UTF-8">
<title>テストページ</title>
<script src="https://code.jquery.com/jquery-3.7.1.min.js" integrity="sha256-/JqT3SQfawRcv/BIHPThkBvs0OEvtFFmqPF/lYI/Cxo=" crossorigin="anonymous"></script>
<style>
.mouseground{
width: 400px;
height: 400px;
background-color: rgb(96, 100, 99);
}
.mousecage{
width: 200px;
height: 200px;
background-color: rgb(69, 143, 124);
}
</style>
</head>
<body>
<div class="main">
<div class="header">
<h1> サンプル </h1>
</div>
<div class="content">
<h2>マウスの移動範囲</h2>
<div class="mouseground">
<div class="mousecage">
</div>
</div>
<div class="desc"></div>
</div>
<div class="footer">
<hr>
<p class="copyright">2019 xxxx all rights reserved.</p>
</div>
</div>
<script>
$(document).ready(function(){
$('.mouseground').on('mouseover', function(){
$('.desc').append('入りました(グレー)<br>');
}).on('mouseout', function(){
$('.desc').append('外れました(グレー)<br>');
});
$('.mousecage').on('mouseover', function(e){
$('.desc').append('入りました(緑)<br>');
}).on('mouseout', function(e){
$('.desc').append('外れました(緑)<br>');
});
});
</script>
</body>
</html>
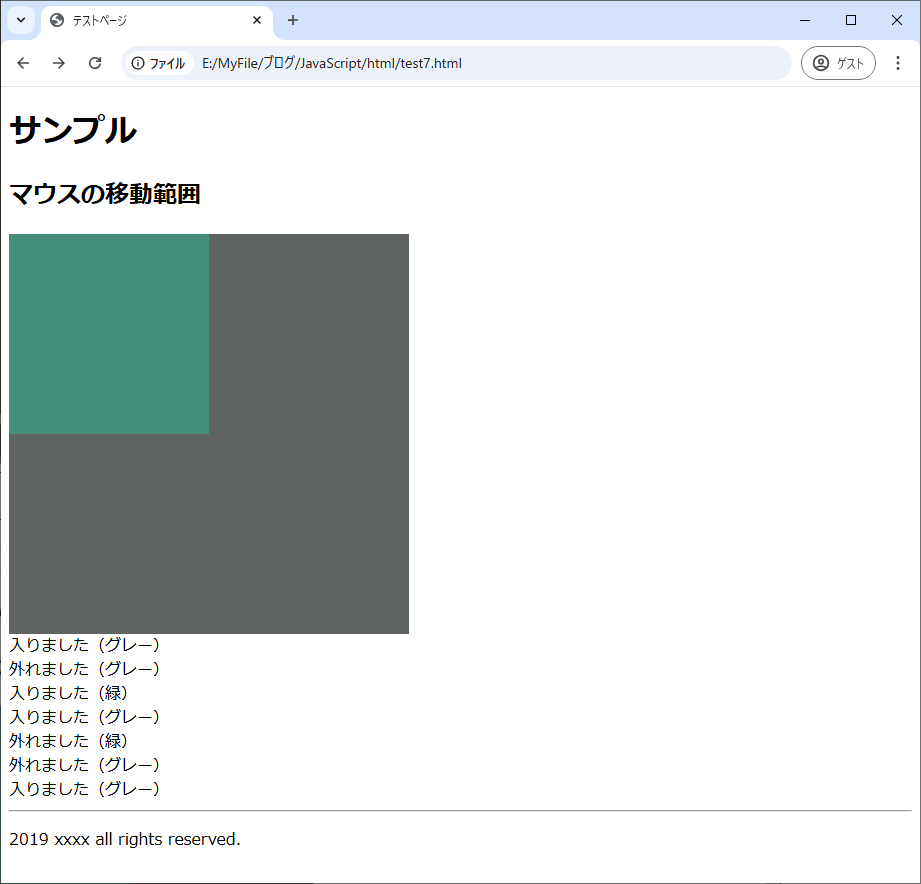